Custom Menu Creation in WordPress: Leveraging register_nav_menus()
For all developers starting to create a website in WordPress, the register_nav_menus()
function is a priority tool. The function allows the creation of custom navigation menus in the theme’s functions file, offering greater flexibility and control over menu management. This function is particularly useful for complex websites that require multiple menus across different locations.
Using register_nav_menus() in functions.php
Here’s a practical example demonstrating how to use the register_nav_menus()
function in function.php to register custom navigation menus:
// Register custom navigation menus
function custom_theme_navigation_menus() {
register_nav_menus( array(
'primary-menu' => esc_html__( 'Primary Menu', 'text-domain' ),
'footer-menu' => esc_html__( 'Footer Menu', 'text-domain' ),
'sidebar-menu' => esc_html__( 'Sidebar Menu', 'text-domain' ),
) );
}
add_action( 'init', 'custom_theme_navigation_menus' );
Detailed Code Breakdown:
- We define a function
custom_theme_navigation_menus()
to register multiple custom navigation menus with unique identifiers and names. - The
register_nav_menus()
function is used to define the locations and labels for each registered menu. - By attaching this function to the
init
action hook, the custom navigation menus will be registered during the initialization of WordPress.
In the admin panel, your new custom navigation menu will look like this:
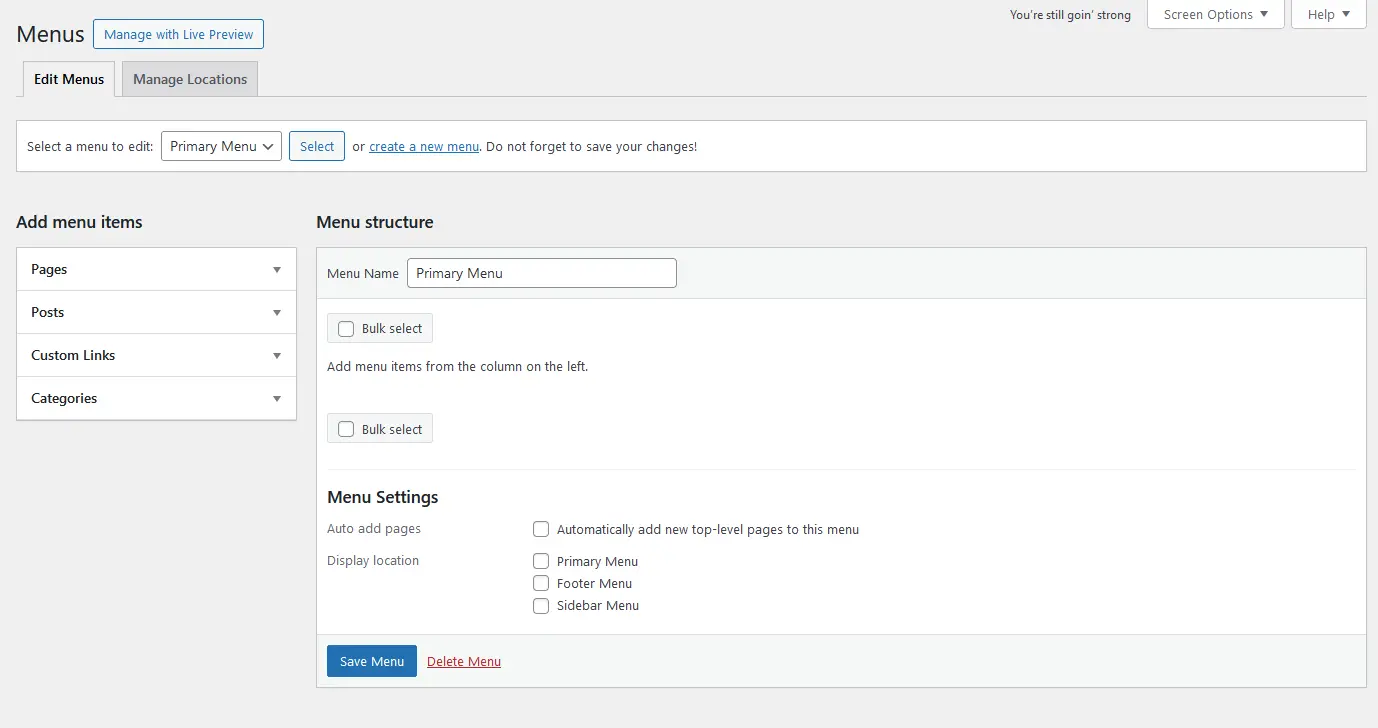
A more complex example of the function’s usage
Here’s a more advanced example of using the register_nav_menus()
function in WordPress, which includes additional features like conditional menu registration based on user roles and theme support checks:
function register_advanced_menus() {
if (current_theme_supports('menus')) {
register_nav_menus(
array(
'header-menu' => __('Header Menu', 'text-domain'),
'footer-menu' => __('Footer Menu', 'text-domain'),
'social-menu' => __('Social Menu', 'text-domain')
)
);
if (current_user_can('editor') || current_user_can('administrator')) {
register_nav_menus(
array(
'editor-menu' => __('Editor Menu', 'text-domain'),
'admin-menu' => __('Admin Menu', 'text-domain')
)
);
}
}
}
add_action('after_setup_theme', 'register_advanced_menus');
Explanation:
current_theme_supports('menus')
: Checks if the current theme supports menus before registering.register_nav_menus()
: Registers multiple menus.'header-menu'
,'footer-menu'
,'social-menu'
: Standard menus for all users.current_user_can('editor')
,current_user_can('administrator')
: Conditional registration for menus available only to users with editor or administrator roles.'editor-menu'
,'admin-menu'
: Special menus for editors and administrators.add_action('after_setup_theme', 'register_advanced_menus')
: Hooks the function to theafter_setup_theme
action, which is triggered after the theme is initialized and set up.
This code snippet demonstrates how to create a dynamic menu structure that adapts to user roles and theme capabilities, providing a tailored experience for different users and ensuring compatibility with the theme’s features.
The interconnection between register_nav_menu() and Walker_Nav_Menu
The register_nav_menus()
function and the Walker_Nav_Menu class in WordPress serve interconnected roles. While register_nav_menus()
enables the registration of multiple menu locations within a theme, the Walker_Nav_Menu
class extends the functionality by providing developers with a way to customize the HTML output of the menus. This class allows for intricate menu structures with unique styling and behavior, making it a powerful tool for developers looking to implement advanced navigation features.
The register_nav_menus()
function is a powerful feature for web developers looking to enhance their WordPress themes with custom navigation menus. By following the steps outlined in this article, you can easily define and manage multiple menus, providing a seamless user experience on your website.